macOS Scripts
You can execute more sophisticated scripts on macOS devices in one of the following languages:
These scripts are sent to devices using the same method as described in Sending Scripts to Devices. Simply create a custom script and copy your script text into the script field.
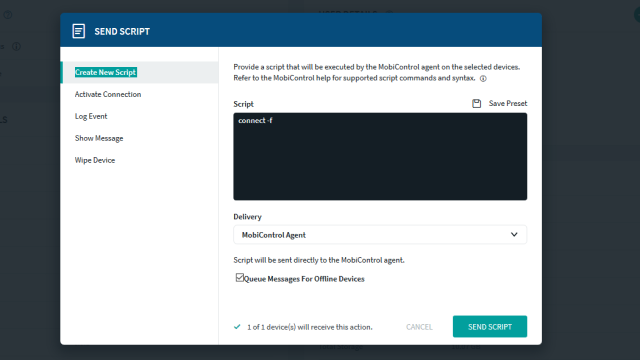
Important: Indicate the script language in the first line on the script code.
BASH Shell
A BASH Shell script example.
shell
a=20
b=10
if [ $a -gt $b ]
then
echo "a is equal to b"
mkdir /Users/a1/Desktop/script/shell1
else
echo "a is not equal to b"
mkdir /Users/a1/Desktop/script/shell
fi
JavaScript
A JavaScript script example
javascript
var myPwl = 1500;
var yourPwl = 1000;
if( myPwl > yourPwl)
{
console.log("My power level is greater than your power level");
var fs = require('fs');
fs.mkdirSync('/Users/a1/Desktop/akash');
}
else
{
var fs = require('fs');
fs.mkdirSync('/Users/a1/Desktop/akash1');
console.log("My power level is less than or equal to your power level");
}
Perl
A Perl script example.
perl
$name = "Ali";
$age = 70;
if ($age >60)
{ mkdir "/Users/a1/Desktop/akashperl", 0755; print "60 plus"; }
else
{ mkdir "/Users/a1/Desktop/akash11", 0755; print "below 60"; }
Python
A Python script example.
python
import os
# define the name of the directory to be created
path = "/Users/a1/Desktop/akash"
try:
os.mkdir(path)
except OSError:
print ("Creation of the directory %s failed" % path)
else:
print ("Successfully created the directory %s " % path)